Chapter 6: Functions (Happy birthday)
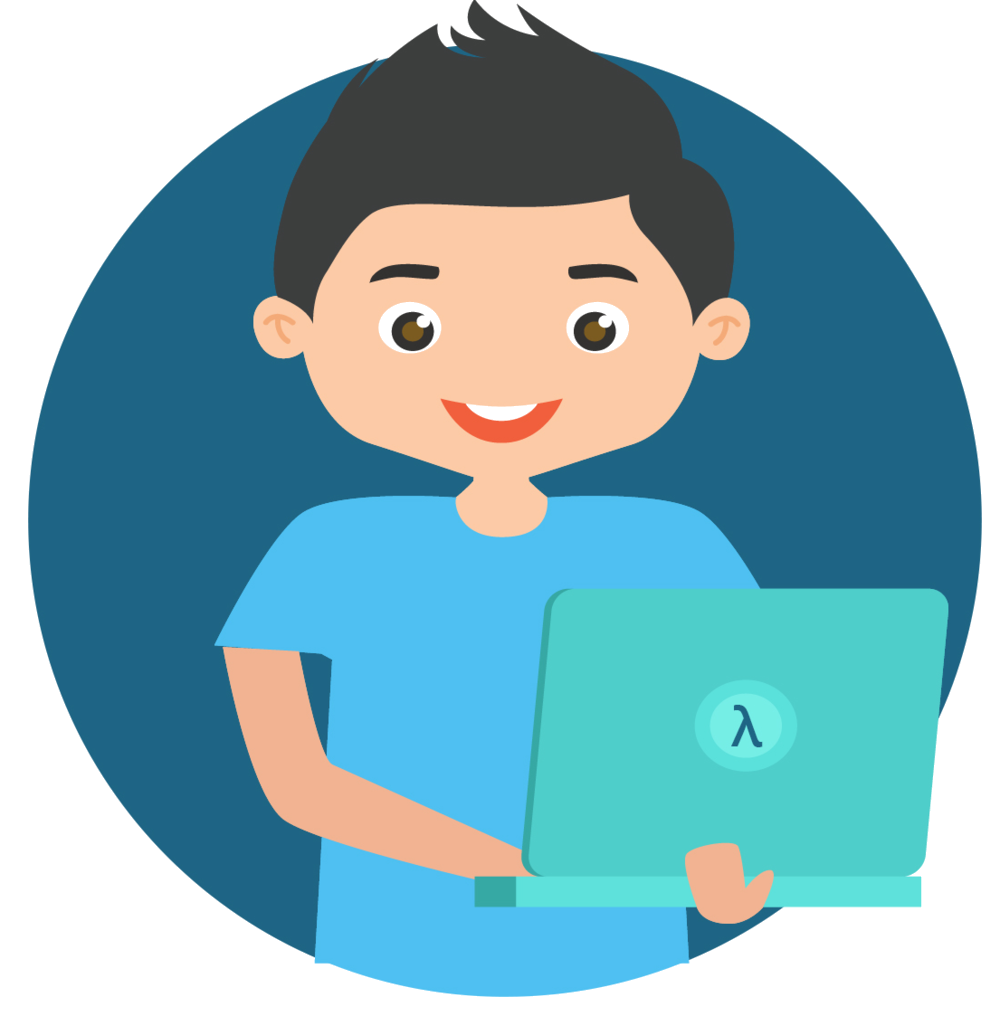
Until now, you have learned how to write expressions using operations like list
, +
, *
, -
, /
and quote
.
Today, you are going to learn how to define your own operations.
There are two kind of operations:
- functions
- macros
In this chapter, we are going to deal with functions.
Why do we need functions?
Let’s say you want to know what is your age in days. It’s simple right? You take your age in years and multiply it by 365 - the number of days in a single year.
If you are 9 years old, your age in days is:
(* 9 365)
And if you have a baby at home, that is 1.5 year old, his age in days is:
(* 1.5 365)
It’s quite annoying to write every time this (* x 365)
stuff. This is why we need functions.
A function is like a formula that you can apply to anything.
In our case, the formula for this calculation is: age-in-years
multiplied by 365.
How to define functions?
Here is how we define a function for the age in days calculation:
#(* % 365)
The structure of function definition is:
- Prepend a
#
(a hashtag) - Insert an expression
- Inside the expression, use
%
the argument to your function
Pay attention: the
#
must be linked to the expression , without any whitespace between them.
As we did with expressions, we can give a name to a function, using def
:
(def age-in-days #(* % 365))
Then we can apply the function to any number:
(age-in-days 9)
(age-in-days 1.5)
It is also possible to apply the function without using its name (it is called an anonymous function):
(#(* % 365) 12)
Do you understand what’s going on in the code snippet above?
Exercises
A. Write a function - named hours->minutes
- that transforms hours into minutes:
()
B. Use hours->minutes
to calculate how many minutes there are in 12 hours:
()
C. Apply the function you defined in #A to 12
, without using its name:
()
D. Write a function - named minutes->hours
- that transforms minutes into hours:
()
E. Use minutes->hours
to calculate how many hours there are in 6000 minutes:
()
F. Apply the function you defined in #E to 6000
, without using its name:
()
Send us a screenshot with your programs to viebel@gmail.com.